[26] Various Improvements
12/12/2020, 10:13pm
Here's an overview of some of the random improvements I've been working on recently. This stuff didn't really add any new gameplay, but a few features were polished and improved.
Minimap Blips
A long time ago I probably mentioned that the minimap was unfinished, missing blips for players and other entities. I finally took some steps to correct that. Check out this blurry image:
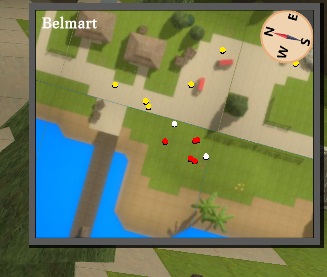
The white dots are players, yellow dots are NPCs, and red dots are items.
I haven't figured out how to fix the weird artifacts on chunk boundaries yet. The minimap tile sprites are not properly snapping to each other, and leave behind those visible seams. Maybe it's some texture filtering issue. I can render the tiles out at a much higher resolution, but I'm avoiding it right now just to keep the game booting up quickly.
As far as I can tell, there is only one task left for the complete minimap implementation, and that would be blips for points of interest (shops, bank, quests, etc). I'll get to it in the future, it doesn't seem too important right now.
Aggro & Combat Indicators
Another cool new thing in the game is aggressive NPCs. I added an AggroComponent which, when added to a combat capable entity, will cause that entity to attack players that wander too close. The entire aggro.rs file itself is less than 100 lines, which is cool. To contrast that, cooking.rs is over 1000 lines. Here's an abridged version of what the meat of the AggroSystem looks like:
for (ent, aggro, combat) in (&ents, &mut aggros, &combats).join() {
// Only check this stuff on an interval
if aggro.range_check_tick_counter < aggro.range_check_tick_counter_target {
aggro.range_check_tick_counter += 1;
continue;
}
aggro.range_check_tick_counter = 0;
if combat.is_in_combat() {
continue;
}
let (position, chunk_coords) = {
// ...
};
let set: BitSet = entities_in_region(chunk_coords);
for (_, tgt_ent, tgt_transform) in (&set, &ents, &transforms).join()
{
let distance = distance(&tgt_transform.position, &position);
if distance < aggro.range {
println!(
"[AggroSystem] Ent {} entered the aggro range of ent {}",
tgt_ent.id(),
ent.id()
);
attack_events.single_write(AttackEvent {
src_ent: tgt_ent,
target_ent: ent,
});
}
}
}
I kind of expected this to cause performance issues, simply due to all the distance checking between NPCs and Players. However, the range checking is staggered and not performed every tick. Also, entities are split into regions and only a single region is checked, so it all seems to be working well. As with everything else in this project, I'll worry about it if it ever actually becomes an issue.
The next thing I added is red circles around NPCs that you are in combat with. This makes it a bit easier to see what is going on, considering there are no real combat animations or other indicators.
Movement, Rotation
Since the beginning, server-side entities in Antorum have had no concept of rotation. I managed to make this work on the client by just predicting everyones look rotation based on their movement. It was never perfect, and then it started failing entirely once I started adding interactable entities like forage nodes (since I wanted to make players face them while interacting). Then, there was the problem of desync across clients. So I quit messing around and just added entity rotations to the protocol and database schema. Now, if you turn one direction and log out, your character will still be looking that direction when you log in. Clients should also stay synced now in terms of which direction a player is facing.
Other
- Tweaked the UI to look a bit nicer (drop shadows, better font sizing)
- While open, the skill guide window now keeps skill levels and exp properly updated
- UI windows no longer block the mouse when dragging to rotate the player camera
- Fishing lines no longer become detached from their poles when the player moves
- Fishing rod item now has a hitbox again
- Tweaked interaction position calcs to avoid players standing on top of NPC shopkeepers
- All starter weapons and armor items have been made more valuable
- All starter resource items have been made less valuable
- Added more client-side error messages for things like an invalid movement or interaction
- Added a comment section to these dev logs
Gonna take a little break and then get back to work. I've got something more interesting in progress for the end of this month.
- Declan (@dooskington)