[27] The Big 2020 End-Of-Year Update
12/30/2020, 10:51pm
This month has been super productive for the game, just because of the holidays. It's not like I'm traveling anywhere this year during my time off work. I finally managed to implement a client-side item cache (same idea as the world cache), as well as make the biggest content update that the game has seen so far. I also dusted off some unannounced stuff that I'm ready to talk about. Overall, I feel like this is a big update to end the year with. Here's an overview of what I got done.
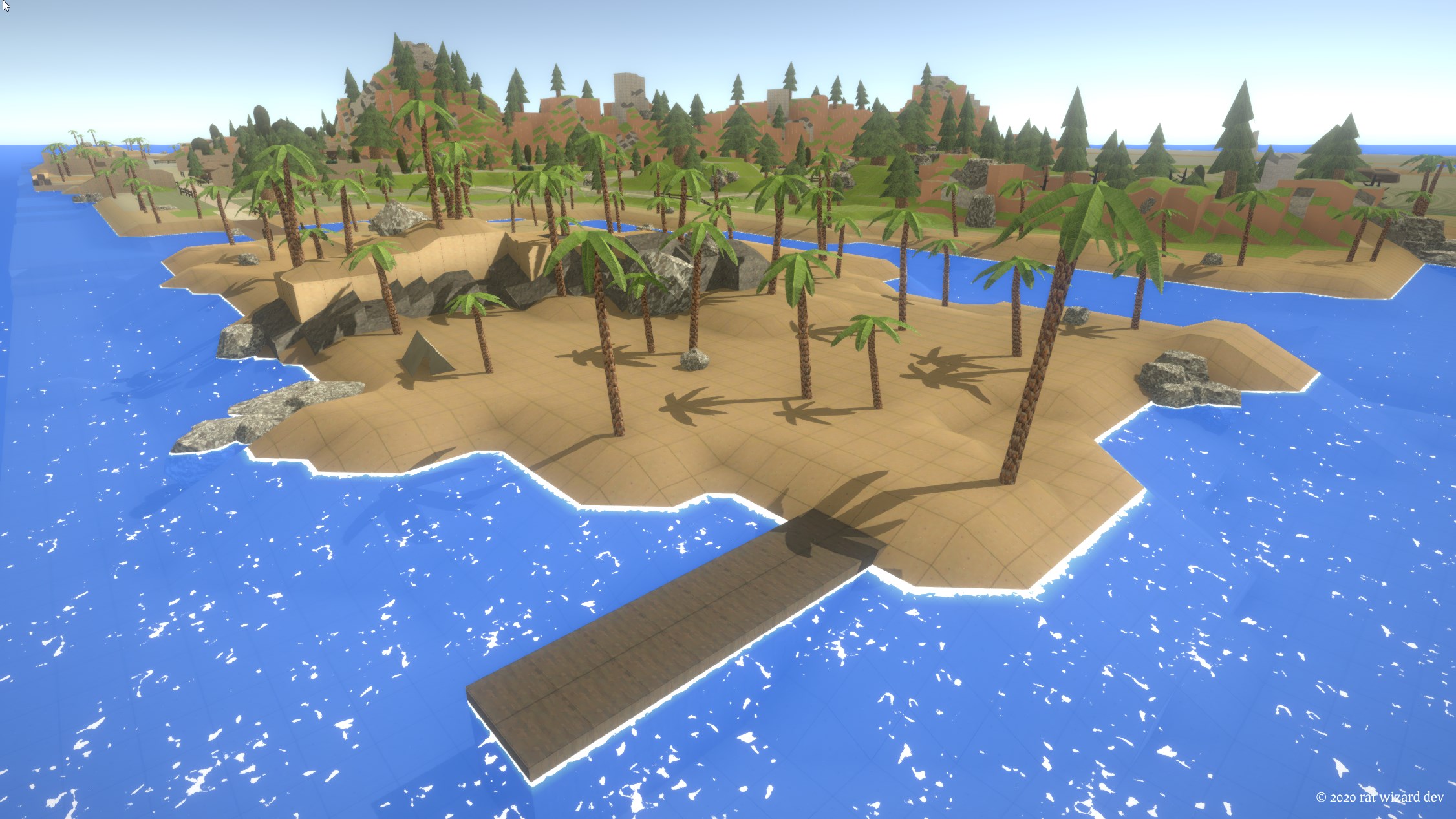
Dialog
The main attraction of this update is Dialog. Players can now talk to NPCs!
Turns out, writing dialog lines is a lot of work and the month didn't have too much time left once I was done with all the code. I'll be adding more as time goes on, but for now there are only a handful of NPCs to talk to. The system is fairly simple, in that dialog cannot effect anything else. Ideally, I'd like to be able to script tiny sequences so that dialog can do things like grant the player items, open user interface windows, and eventually, mutate quest state.
Dialogs are defined in .toml files. Here is a segment of one:
entry_node_id = 0
[[nodes]]
type = "Lines"
id = 0
text = ["Hail, Traveller. Keeping your nose out of trouble?"]
next_node_id = 1
[[nodes]]
type = "Responses"
id = 1
[[nodes.options]]
text = "Where am I?"
next_node_id = 2
[[nodes.options]]
text = "Where can I purchase supplies?"
next_node_id = 4
[[nodes.options]]
text = "Goodbye."
next_node_id = -1
[[nodes]]
type = "Lines"
id = 2
text = ["You are in Belmart. It's a fishing town and trade hub."]
next_node_id = 3
...more nodes
This whole system is networked via three tiny packets. After all, it's just text being sent one direction, and response selections being sent the other.
/// 58 - 0x3A - Dialog
/// Server
DialogPacket {
pub ent_net_id: u64,
pub lines: Vec,
pub response_options: Vec,
}
/// 59 - 0x3B - Dialog Option Request
/// Client
DialogOptionRequestPacket {
pub option_idx: u8,
}
/// 60 - 0x3C - End Dialog
/// Server
DialogEndPacket;
NPCs that are in a conversation won't "squak" random sayings, and they won't wander around. They also cannot be attacked. I figured it would be annoying to have NPCs get killed by other players while you were talking to them. To compliment this, players also can't start a conversation with an NPC that is in combat.
I like how NPCs actually move and turn to face you when you talk to them. That is a pretty concise bit of code from the DialogSystem, so I can share it here:
// ... handle dialog and determine 'lines' and 'response_options'
let packet = DialogPacket {
ent_net_id: tgt_ent_net_id,
lines,
response_options,
};
pckt_events.send(PacketPlayerRecipient::Player(player.id), packet);
// Make the target entity move to the center of their occupied tile, as well as face the player.
let (target_conversation_position, target_conversation_rotation) = {
let src_transform = transforms.get(*src_ent).unwrap();
let tgt_transform = transforms.get(*tgt_ent).unwrap();
let tgt_tile = map::world_coords_to_tile_coords(&tgt_transform.position);
let tgt_tile_center_pos = map::tile_coords_to_world_coords(&tgt_tile)
+ Vector2f::new(TILE_SIZE / 2.0, TILE_SIZE / 2.0);
let dir = (src_transform.position - tgt_tile_center_pos).normalize();
let rot = Point3f::new(0.0, f32::atan2(dir[0], dir[1]).to_degrees(), 0.0);
(tgt_tile_center_pos, rot)
};
move_events.single_write(MoveEvent {
src_ent: *tgt_ent,
destination: target_conversation_position,
final_rotation: Some(target_conversation_rotation),
start_tick: ticks.0,
should_end_combat: true,
})
Client-side Item Cache
So far, each time I added an item, I also needed to update a file on the client so there was some client-side knowledge of it. This was a massive pain, and kept me from adding new items regularly. I'm happy to say this is no longer the case, and I finally got around to giving items the same treatment that the world/terrain got. They are now downloaded by the client when outdated, so any changes to the item database are propagated to all players automatically.
The coolest bit about this is the item icons. Now, after being downloaded, the item cache has all item icons automatically generated. This all works pretty much the same way as the minimap tile rendering does.
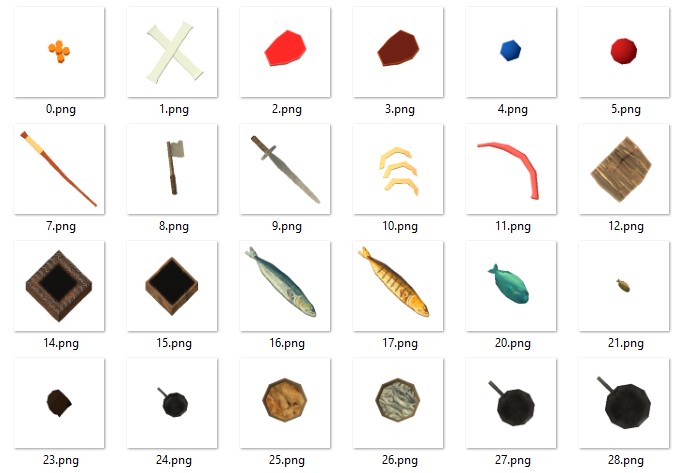
There is plenty of room for improvement. I'd like to add some outlines to the items (or maybe drop shadows, like items had in Morrowind?), and the render camera needs to adjust its size based on the bounds of the item model. You can probably see that item 21 (Cooked Blue Skipper) is looking a bit too tiny in its item icon.
Better Inventory
The inventory is feeling much better these days. Now you can actually drop more than one item at a time, through the use of the handy dandy new right-click menu. You can also left click to quickly equip/unequip, eat, or interact with items.
I don't love working on user interface elements. This particular one was a gauntlet of bug fixes and edge cases, and then all the interactions had to be properly sent to the server. I'm glad to be done with this, I spent a lot of time making sure it was actually usable and I hope I don't have to touch it for a while.
Better Dropped Items
This is a simple but cool fix. When dropped on the ground, equipment items didn't really look right, because their models were meant to be placed on a characters body. However, it is now possible to specify an model id override for when an item is dropped on the ground.
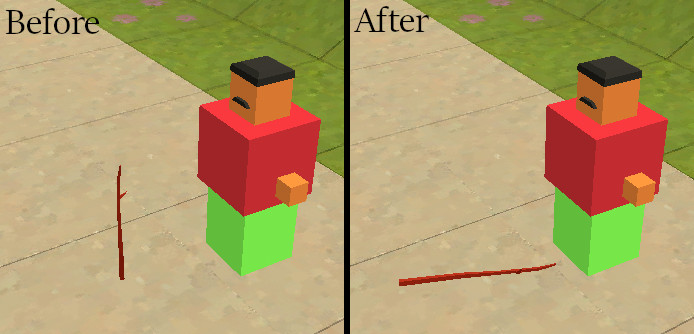
Along with this, all item hitboxes were improved. No more pixel-hunting to pick up items!
Better Bartering
A lot of the inventory improvements that I just talked about also applied to the barter window. However, an added bonus here is that you can now sell all of a certain item in bulk, even if that item isn't stackable. This makes shop trips much less tedious.
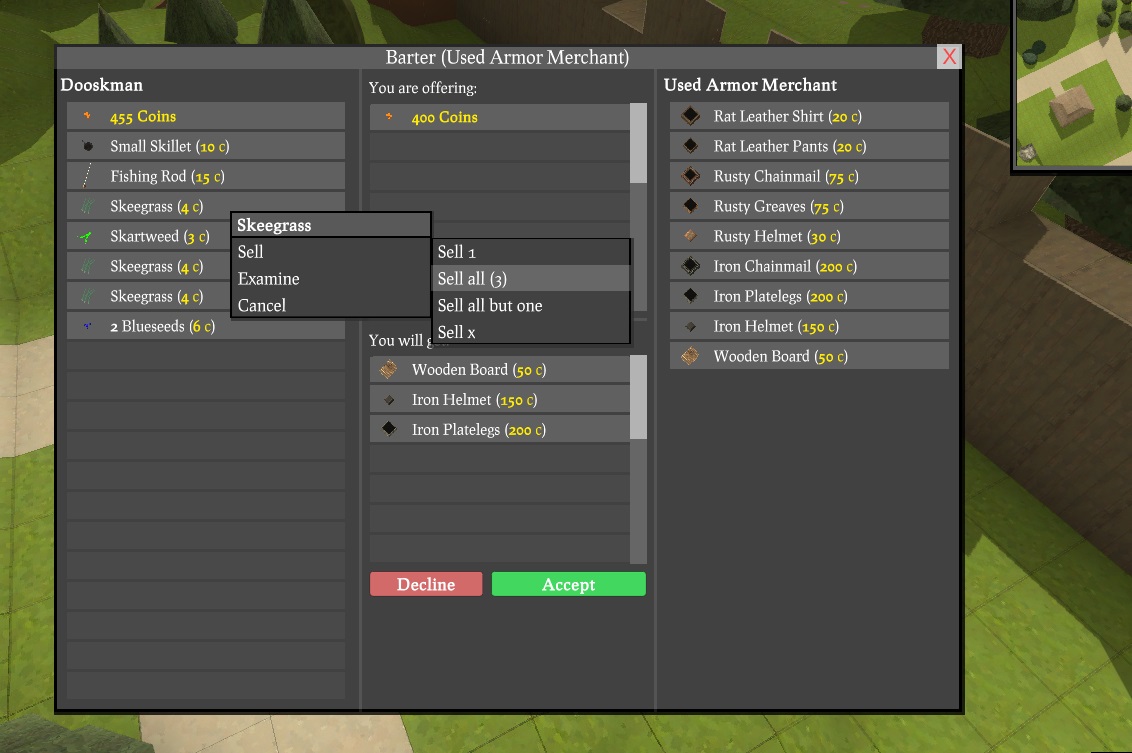
This whole menu still needs work to look nicer, but that's low on my priority list.
Better Minimap Quality
I mentioned the blurry minimap in the last dev log. Turns out, I was accidentally saving the minimap tiles at a much lower resolution than they were originally rendered. So, they looked great on the first run of the game, but subsequent runs were loading up the low quality versions of each tile. I fixed that up pretty quickly, and we are back in business:
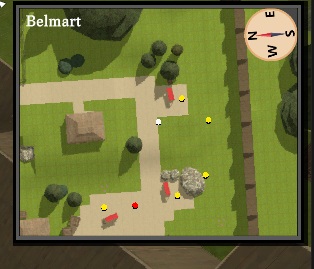
Better Main Menu and Options Menu
The main menu has gotten some love, and I also added an options menu!
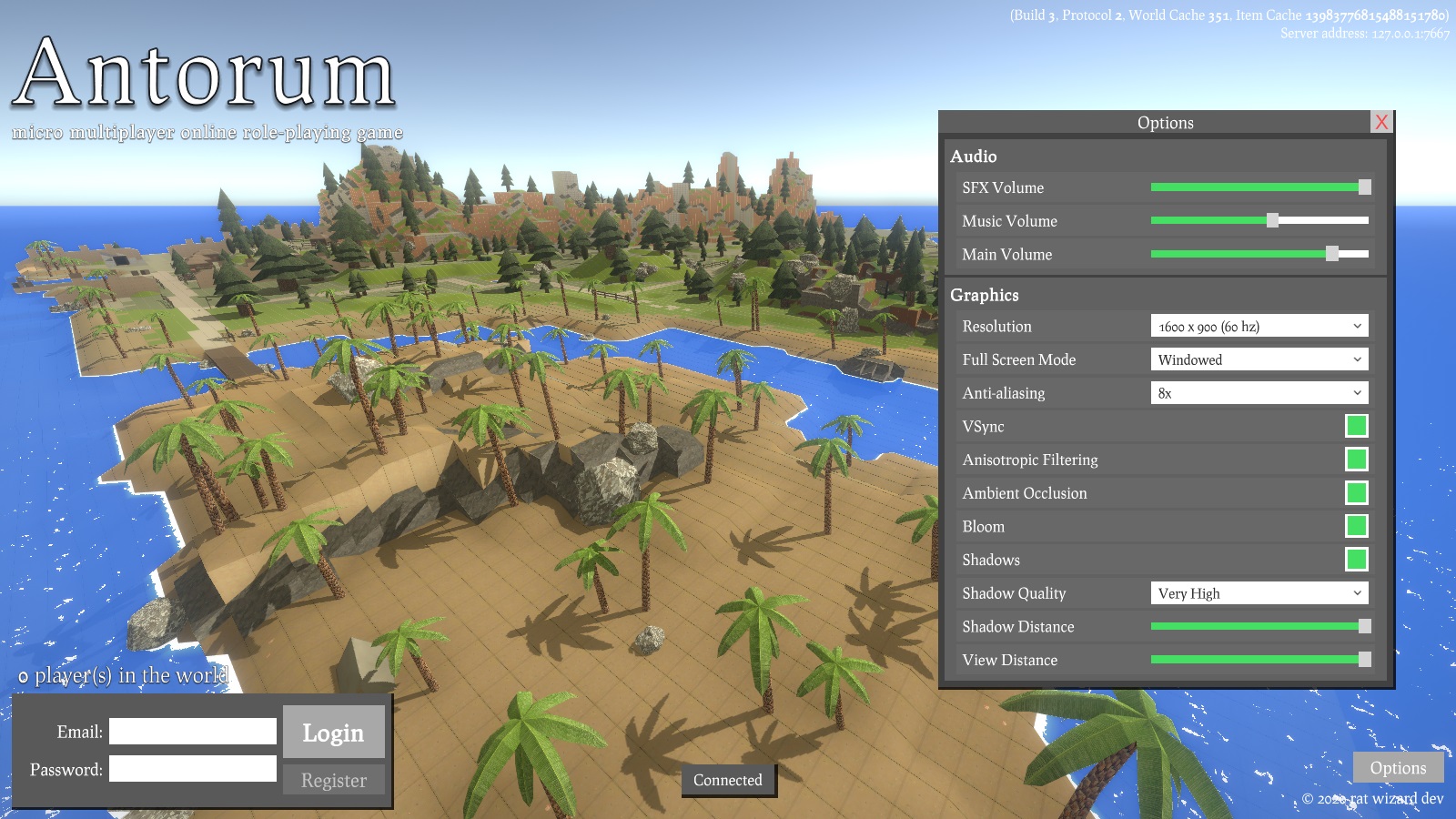
You may have already noticed all the cool new post-processing effects in the game - I got a little carried away. Then I realized that if the effects were in the game, you needed to be able to turn them off. So, I got sidetracked for a while ironing out this menu. It is also accessible in-game.
Not pictured here is the resource download screen, which also shows a view of any in-progress item/minimap rendering.
More Fish, More Plants
I added a few new fish, junk fishing items, and plants to the world. Here are some of them:
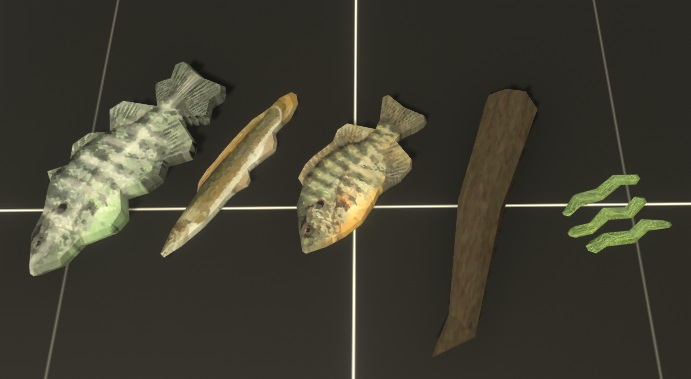
Along with the new fish are a few new cooking recipes.
More Equipment
I also added some new armor sets and weapons. Here's a small preview of some of those items:
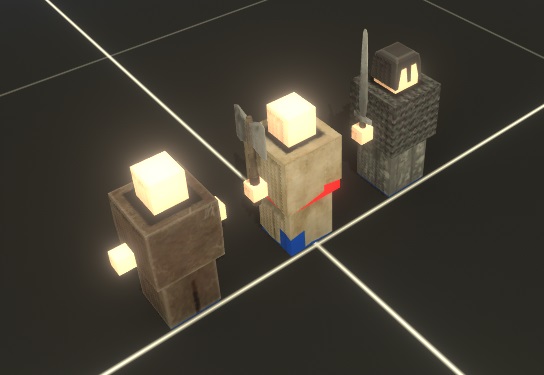
Some can be purchased at shops, while others are only dropped from certain NPCs.
The Graveyard
This is the part I am most excited about. I spent a long time designing and building this zone, and it is the most challenging one in the game yet. Actually, I'm not even sure it can even be explored very easily (alone, at least) with any currently accessible gear. My bad, I have a lot of balance work to do in general.
Here, players will find new plants to forage, monsters to fight, and loot to acquire. There may even be a new hidden and rare fishing spot...
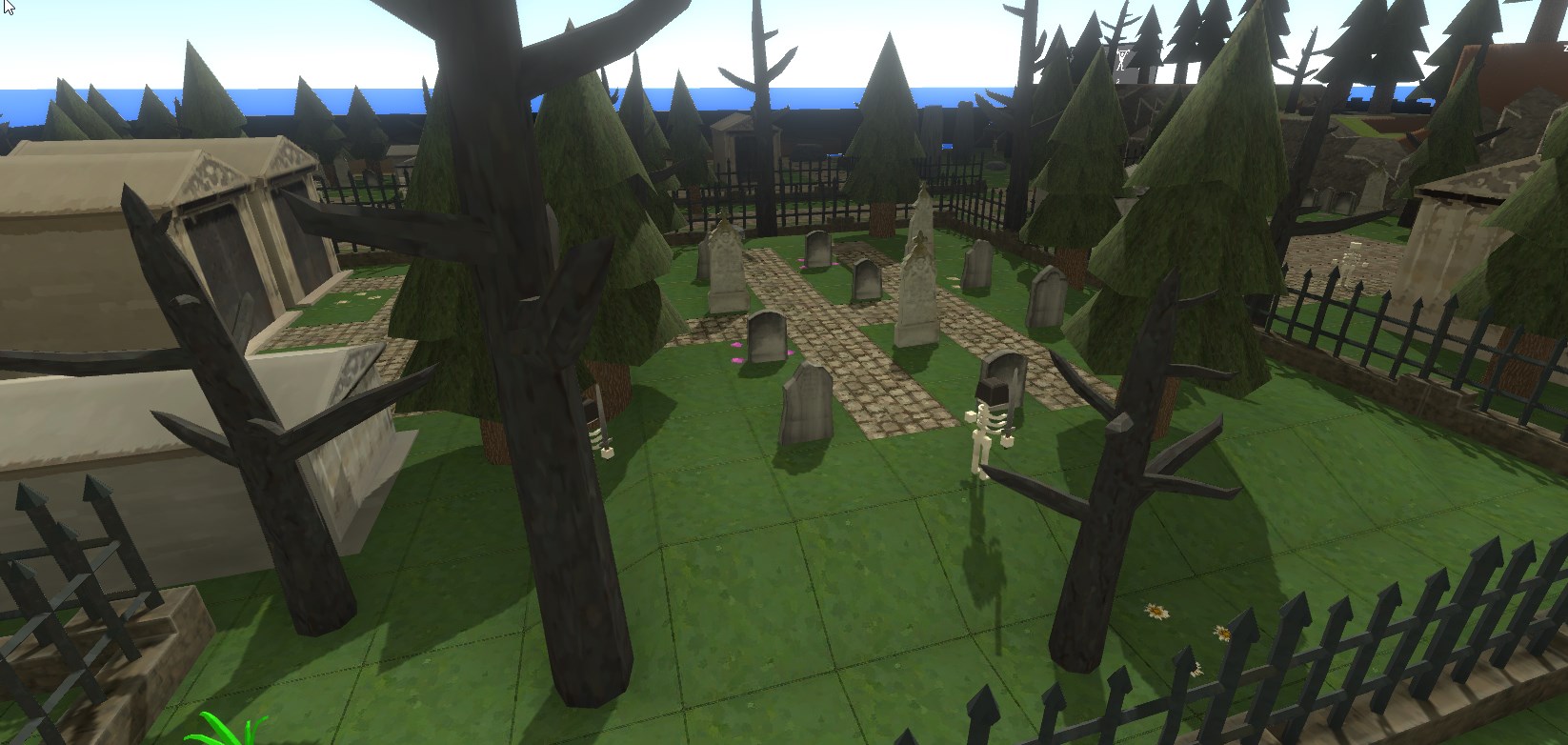
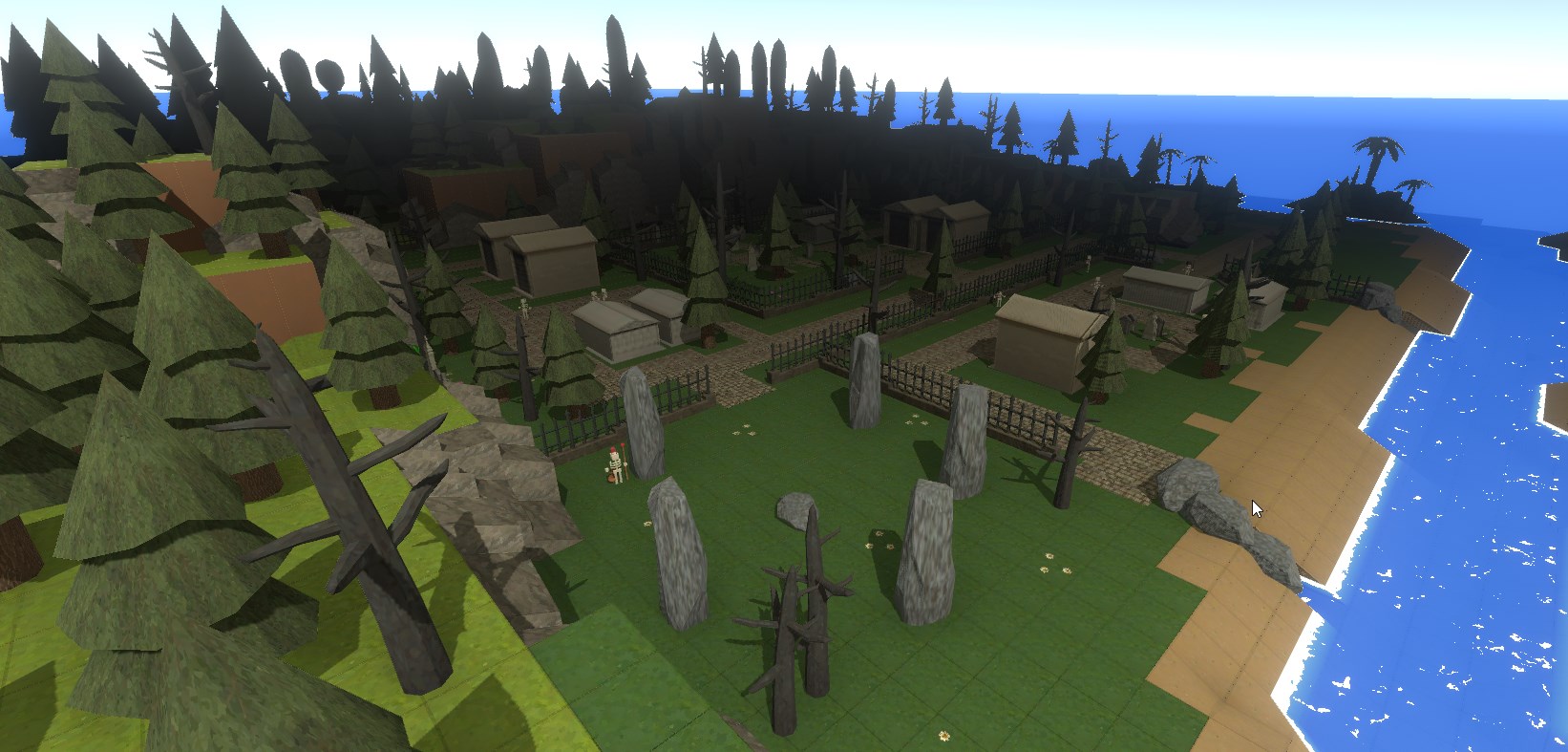
My favorite part of this zone is the new boss:
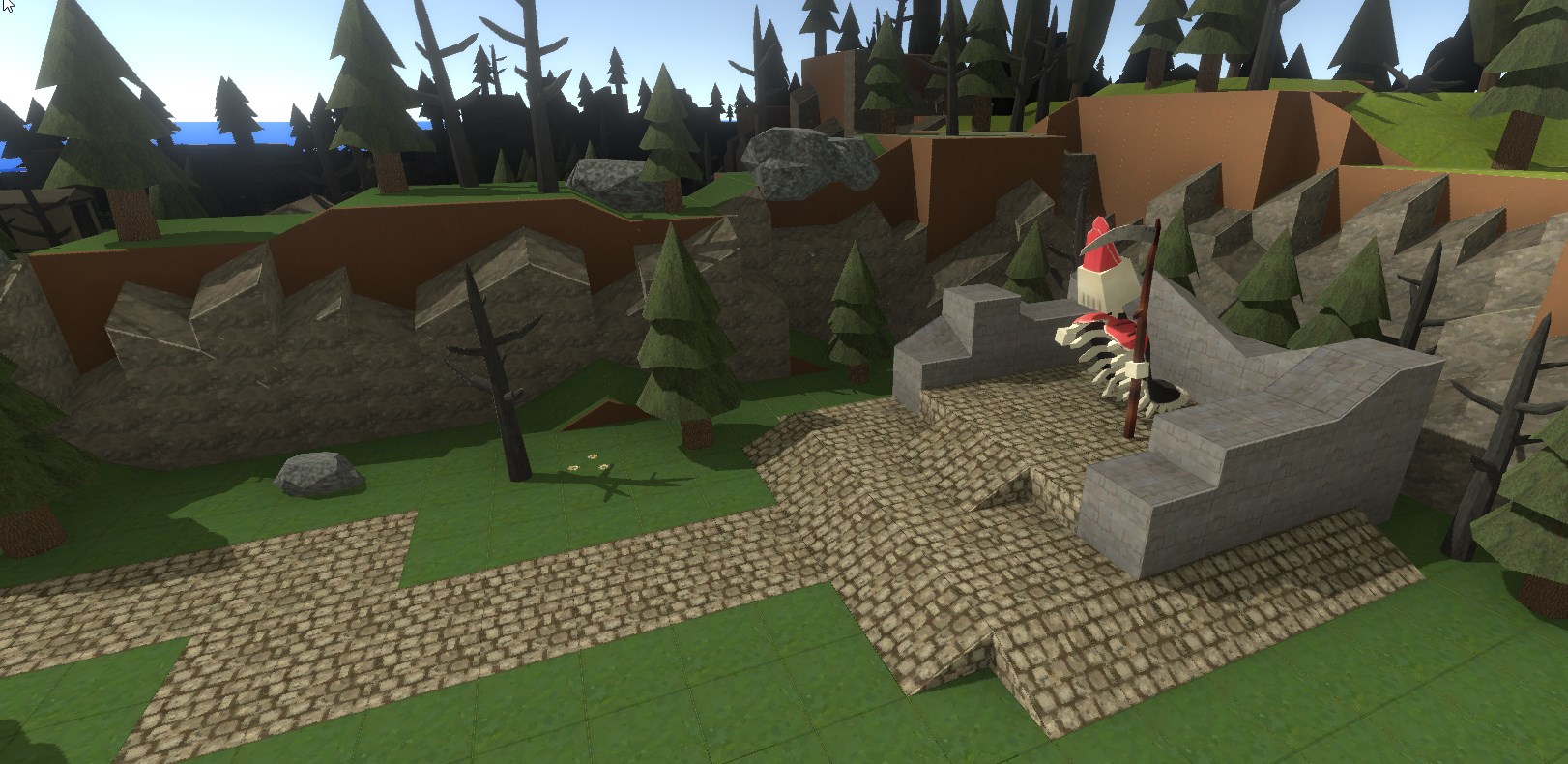
The scythe is a rare drop, and currently the best weapon in the game. If you are one of the very few people who are playing the game, and are looking for a challenge, head out of Belmart via the North road. Follow the road past the woodlands, then at the fork, go left. You'll probably want to try and purchase some iron armor, and stock up on food, before you make the quest.
Music and Audio
Some things are stirring in this area. Hope to have more updates soon.
Other
- Fixed server crash when a player tried to chat on the same tick that they respawned
- Fixed many bugs with inventory item dragging
- Added more messages from server to alert player when they have done something unsupported or wrong (such as trying to move to an incorrect destination)
- Added more colors to chat user interface to make it more clear when players are chatting
- Added over ten new entities
- Added over twenty new items
- Added two new undisclosed bosses to the world
I know I said I would announce a playable alpha before the end of the year, but the game still isn't ready. I've shared it with a few friends though, and I'm trying to incorporate as much feedback as I can while still completing new game features.
In total, all this content required that I make over 60 new 3D models, so I really have been getting a lot of art practice in. I also realized an early mistake I had made in my pipeline over the year, and had to go back and update 90 models by hand to be compatible again. Despite my best efforts, it is still hard to keep track of every little thing I change in the game. So, I probably haven't captured everything here. I really wanted to finish up Banking this month, but it didn't make the cut - I think that will be the first thing I post about in 2021. Thanks for reading this year. Here's to another one!
- Declan (@dooskington)